Introducing the Const Statement
The Const statement declares constants. The header image above shows the Const statement’s syntax. Moreover, based on its location in code, it can confer different levels of scope on variables and objects.
The image below shows the different scope levels in VBA. Also, we’ve delved into scope levels in a separate article, make sure to check it out if you need a refresher.
![VBA Program Identifiers – Scope Levels [from the narrowest (block level) to broadest (application level)]](https://masterofficevba.com/wp-content/uploads/2020/09/VBA-Program-Identifier-Scope-Levels-1024x563.png)
The Const statement may be placed in blocks or procedures, or it can sit atop modules (outside all procedures). In each case, it confers block, procedure, module, or project level scope on a variable or object, respectively.
Salient Points on the Const Statement
There are several vital things to note about the Const statement:
- A module-level variable’s scope (which is private by default) is editable. But you can’t alter a block-level or procedure-level variable’s scope. In fact, the Private and Public keywords are legal only at the module-level. However, this only applies in Standard modules, in Class modules a constant’s scope (private by default) is immutable.
- You can include the Private or Public keyword in a module-level constant’s declaration, but not both. That’s in addition to the Const keyword which must always be present.
- The Const and Private keywords have the same effect at the module-level. However, adding the Private keyword to the Const keyword improves code readability.
- A single Const statement can declare several constants. To do this, insert a comma after each constant’s name (or data type, if specified). In this use-case, a Private or Public keyword applies to all the constants in the statement. Moreover, if a constant’s data type is set, then that of all other constant’s in the statement must also be set.
- You can omit a constant’s data type in its declaration. In that case, the compiler assigns the most appropriate data type to it. However, the compiler does not assign the variant data type to such a constant, as it does for the Dim statement.
- Lifetime does not apply to constants. So, do not replace the Const keyword with the Static keyword. That would result in a compilation error.
Sample Code (Examples)
The sample code below shows the Const statement in use. It also illustrates the salient points discussed above.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
Option Explicit 'declare constants accessible only to procedures in the current module Const str_NamesCategory As String = "Nordic" Private Const int_CountryCode As Integer = 971 ' clearly, adding PRIVATE is more readable than using just CONST 'declare constants accessible to procedures in/out-side the current module Public Const str_SelectionCriteria As String = "Education" ' Sub Const_Statement_Procedure_Level_Examples() 'declare a procedure-level constant with data type Const int_Age As Integer = 33 ' expectedly, arrays and objects cannot be mapped to constants 'without data type, the compiler asigns the most appropriate data type Const var_Age = 33 Const var_CarBrand = "Tesla" ' see the most apt types assigned in the Immediate Window Debug.Print "var_Age's Type is " + Str(VarType(var_Age)) Debug.Print "var_CarBrand's Type is " + Str(VarType(var_CarBrand)) 'declare multiple procedure-level constants in one Const statement Const int_CountPens As Integer = 5, var_GenderMale = True ' see the most apt type assigned in the Immediate Window Debug.Print "var_GenderMale's Type is " + Str(VarType(var_GenderMale)) End Sub |
When you run the sample code above, the VarType function (lines #22-23 and #28) returns the data type of the variable passed to it as an argument. Those function return-values are then shown in the Immediate window using the Print method of the Debug object, as shown below.
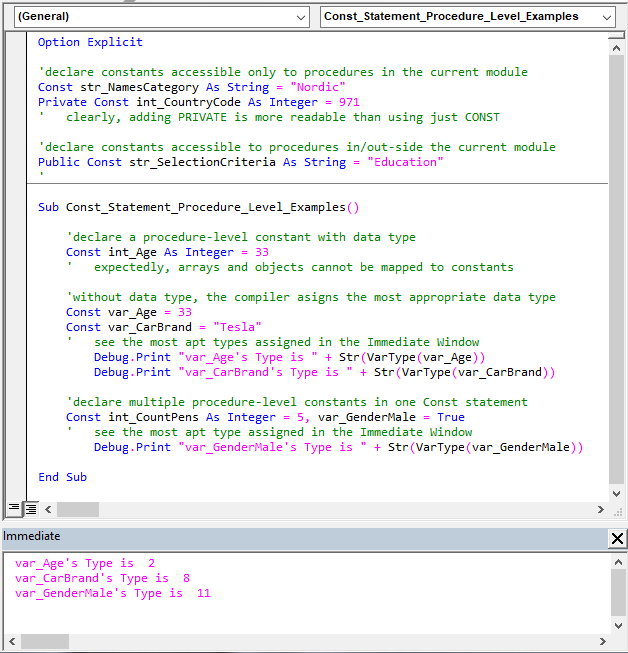