Introducing the If Statement
Humans often decide on a course of action by evaluating one or more criteria. Likewise, computer programs select a flow of execution (a.k.a. control) by evaluating expressions. The If-Then-[ElseIf]-[Else] statement is one of two VBA selection statements (the Select Case statement being the other).
An execution path is only chosen if the expression evaluates to a specific Boolean value. The Boolean return is either True or False, which equates to a non-zero (≠ 0) or zero (= 0) value. In this sense, the If-Then-[ElseIf]-[Else] statement is also called a conditional statement.
The header image above shows the If-Then-[ElseIf]-[Else] statement’s syntax. Also, the flowchart below illustrates its logic flow.
![Flowchart showing the If–Then–[ElseIf]–[Else] statement’s logic flow](https://masterofficevba.com/wp-content/uploads/2021/02/Flowchart-showing-the-If–Then–ElseIf–Else-statements-logic-flow.png)
The single-line form has the same elements as the block-form (header image). However, all its elements are on a single line with colons separating multiple statements.
Also, in the single-line form the first set of statements (i.e., #1) is not optional and the End If statement is invalid. You should use the single-line form for short and simple selections, instead of the block form.
Salient Points on Usage
There are several important things to note about the If–Then-[ElseIf]-[Else] statement:
- Control expressions can be of two types, numeric or string and TypeOf–Is expressions. In either case, the expression evaluates to a True (i.e., ≠ 0) or False (i.e., = 0) value.
- The compiler treats a Null control expression return as False.
- The block form is more versatile and easier to read, maintain, and debug than the single-line form.
- In the block form, If statements can be nested within preceding If, ElseIf or Else statements. But each nesting must have its own End If statement. Also, you can use as many ElseIf statements as needed, but none can appear after the Else statement – unless as part of a new nested If statement.
- You can include Line labels or line numbers in the If-block. But the If statement must be the first entry on the block’s first line.
- Although the Else statement is optional, you should always include it. That way you control what happens should all control expressions in the preceding If and ElseIf statements return False. Otherwise, program execution (or flow of control) shifts to the line below End If.
Too many nested If-Then-[ElseIf]-[Else] statements make your code difficult to read. When different values of an expression lead to different execution paths, use the Select Case statement instead.
Sample Code (Examples)
The code samples below show the various forms of the If-Then-[ElseIf]-[Else] statement in use. They also illustrate the crucial points discussed above.
In each sample code, the aim is to decide a person’s generational class based on their birth year. The programs adopt the Pew Research Center’s definition (shown in the image below).
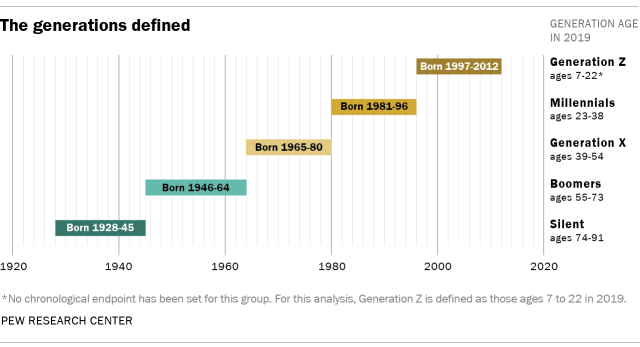
Single-line If-Then Statement
The sample code below illustrates the single-line If-Then statement’s usage. It assigns age-based generations as per the Pew Research Center’s definition.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 |
Option Explicit Public Sub If_Statement_Example01() 'define generations using single-line if-statements 'declare variables Dim int_BirthYear As Integer, str_Validation As String, str_Gen As String 'get individaul's birth year via VBA's built-in InputBox function int_BirthYear = CInt(InputBox("Enter birth year, YYYY", _ "Defining Generations")) 'validate the provided birth year against the applicable year range If int_BirthYear < 1928 Then str_Validation = "Invalid birth year" If int_BirthYear > 2012 Then str_Validation = "Invalid birth year" If str_Validation = "Invalid birth year" Then MsgBox str_Validation If str_Validation = "Invalid birth year" Then Exit Sub 'birth year is valid, now assign generation If int_BirthYear >= 1928 And int_BirthYear <= 1945 Then str_Gen = "Silent" If int_BirthYear >= 1946 And int_BirthYear <= 1964 Then str_Gen = "Boomer" If int_BirthYear >= 1965 And int_BirthYear <= 1980 Then str_Gen = "Gen-X" If int_BirthYear >= 1981 And int_BirthYear <= 1996 Then str_Gen = "Millennial" If int_BirthYear >= 1997 And int_BirthYear <= 2012 Then str_Gen = "Gen-Z" 'display the result to the user via VBA's built-in MsgBox function MsgBox "A person born in " & CStr(int_BirthYear) & _ " is part of the " & str_Gen & " generation.", vbOKOnly, _ "Defining Generations" End Sub |
In brief, the program:
- Declares Integer and String variables int_BirthYear, str_Validation, and str_Gen (code window line #8). These variables store the user’s birth year of interest, an input validation message, and the defined generation.
- Collects the user’s birth year of interest via the CInt and InputBox functions (line #11).
- Certifies that the user’s birth year of interest is within the applicable range (lines #15-18). Otherwise, it ends execution (line #18). The single-line If-Then statement, MsgBox function, and Exit Sub statement are used for this.
- Assigns a generation to the birth year by matching it to the Pew Research Center’s definition. Single-line If-Then statements and the And operator are used for this (lines #21-25).
- Displays the result via the MsgBox function (lines #28-30). Also, with the line continuation character (underscore, _), the function is able to span across several lines.
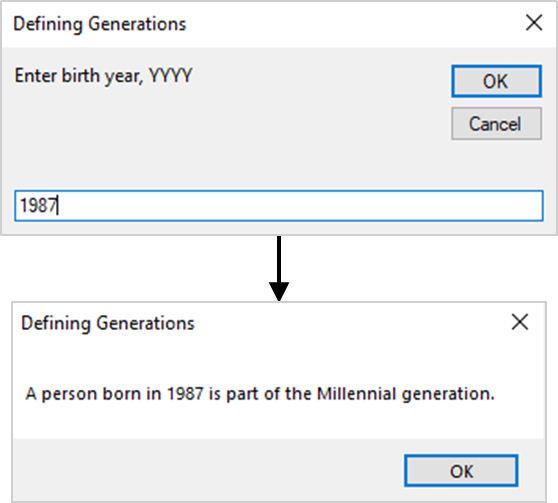
CInt and CStr are built-in functions for converting expressions into Integers and Strings. However, CInt throws an error if the user enters non-numeric input, so the Val function would be a better choice here.
The InputBox and MsgBox functions are also built-in VBA functions. They serve as means of inputting and outputting data to and from programs.
Block-form If-Then-[ElseIf]-[Else] Statement
The single-line If–Then statement’s sample code above works, but it is inefficient. The issue is that each If–Then statement in the generation assignment block must run. But the birth year can only ever be in one age-range at any time, so only one of these If–Then statements is useful at any one time.
The If–Then-[ElseIf]-[Else] statement’s block form stops range-checking once it finds a match. The sample code below shows its use in assigning generations as per the Pew Research Center’s definition.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
Option Explicit Public Sub If_Statement_Example02() 'define generations using block-form if-statements 'declare variables Dim int_BirthYear As Integer, str_Gen As String 'get individaul's birth year via VBA's built-in InputBox function int_BirthYear = Val(InputBox("Enter birth year, YYYY", _ "Defining Generations")) 'validate the provided birth year against the applicable year range If int_BirthYear < 1928 Or int_BirthYear > 2012 Then MsgBox "Invalid birth year", vbOKOnly, "Defining Generations" Exit Sub End If 'birth year is valid, now assign generation If int_BirthYear >= 1928 And int_BirthYear <= 1945 Then str_Gen = "Silent" ElseIf int_BirthYear >= 1946 And int_BirthYear <= 1964 Then str_Gen = "Boomer" ElseIf int_BirthYear >= 1965 And int_BirthYear <= 1980 Then str_Gen = "Gen-X" ElseIf int_BirthYear >= 1981 And int_BirthYear <= 1996 Then str_Gen = "Millennial" Else str_Gen = "Gen-Z" End If 'display the result to the user via VBA's built-in MsgBox function MsgBox "A person born in " & CStr(int_BirthYear) & _ " is part of the " & str_Gen & " generation.", vbOKOnly, _ "Defining Generations" End Sub |
Nested If-Then-[ElseIf]-[Else] Statement
You can improve the structure of the block-form If–Then-[ElseIf]-[Else] statement’s sample code above by nesting. The sample code below shows the nested If–Then-[ElseIf]-[Else] statement’s use in defining generations as per Pew Research Center’s classification.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 |
Option Explicit Public Sub If_Statement_Example03() 'declare variables Dim int_BirthYear As Integer, str_Gen As String 'get individaul's birth year via VBA's built-in InputBox function int_BirthYear = Val(InputBox("Enter birth year, YYYY", _ "Defining Generations")) 'validate the provided birth year against the applicable year range If int_BirthYear < 1928 Or int_BirthYear > 2012 Then 'birth year is not valid, warn user and end procedure MsgBox "Invalid birth year", vbOKOnly, "Defining Generations" Exit Sub Else 'birth year is valid, now assign generation If int_BirthYear >= 1928 And int_BirthYear <= 1945 Then str_Gen = "Silent" ElseIf int_BirthYear >= 1946 And int_BirthYear <= 1964 Then str_Gen = "Boomer" ElseIf int_BirthYear >= 1965 And int_BirthYear <= 1980 Then str_Gen = "Gen-X" ElseIf int_BirthYear >= 1981 And int_BirthYear <= 1996 Then str_Gen = "Millennial" Else str_Gen = "Gen-Z" End If End If 'display the result to the user via VBA's built-in MsgBox function MsgBox "A person born in " & CStr(int_BirthYear) & _ " is part of the " & str_Gen & " generation.", vbOKOnly, _ "Defining Generations" End Sub |
Here, the generation assignment block (lines #19-29) nests beneath the input validation block. Also, the assignment block now has an Else statement after the Exit Sub statement (line #17).