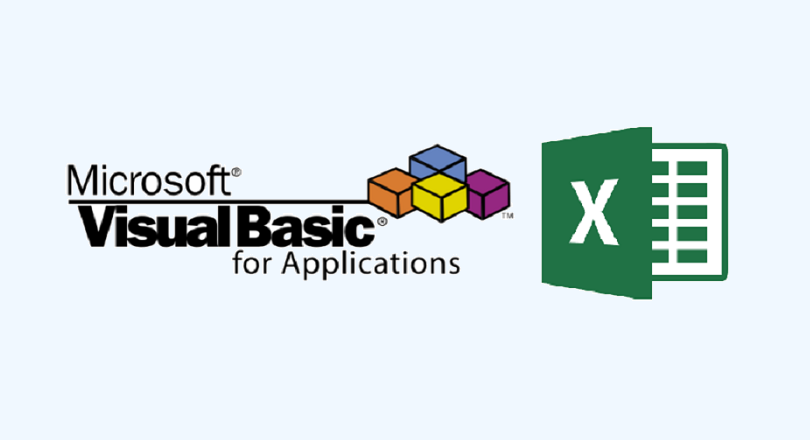
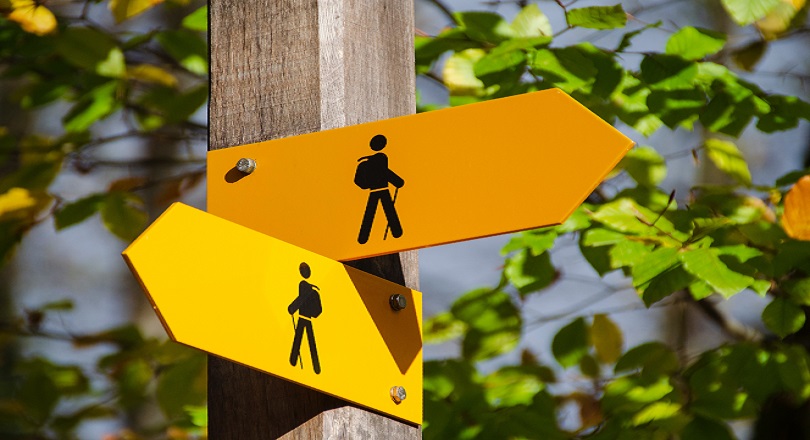
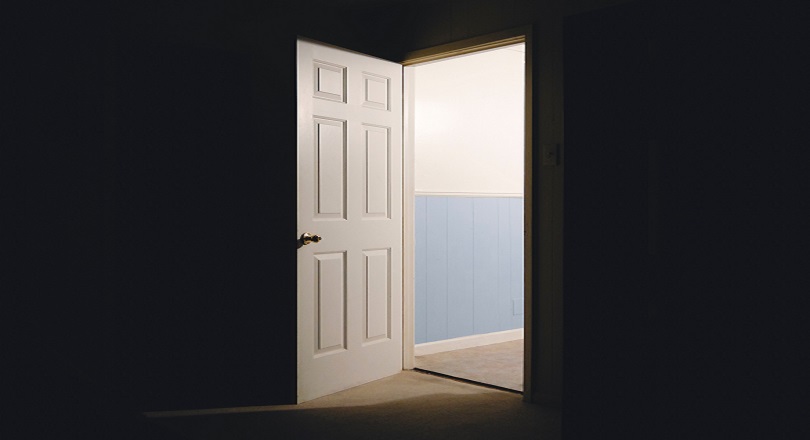
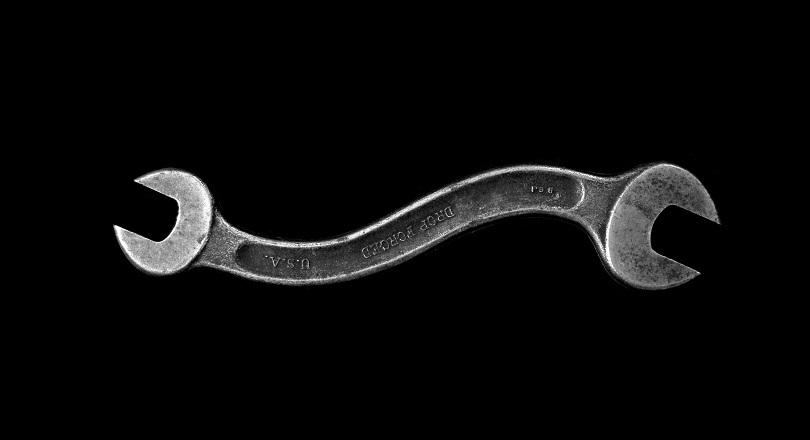
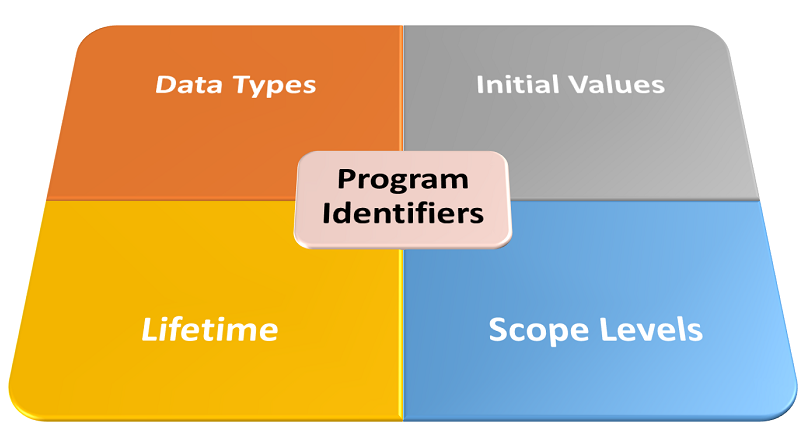
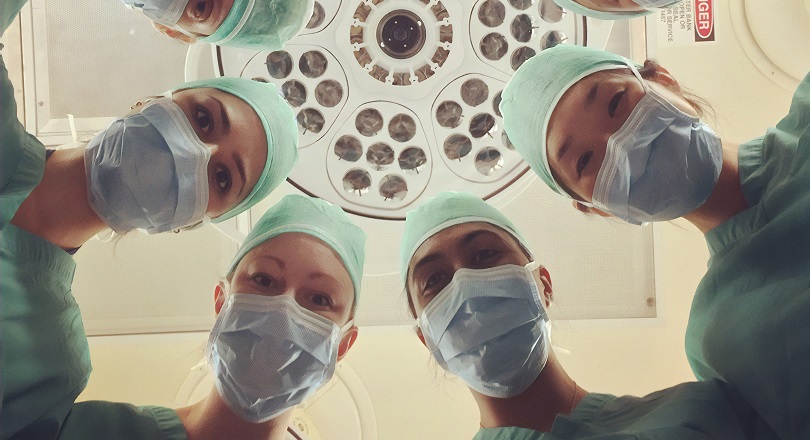
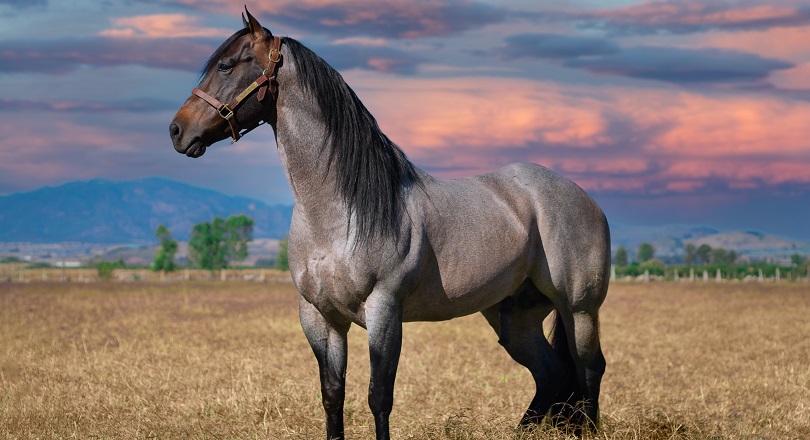
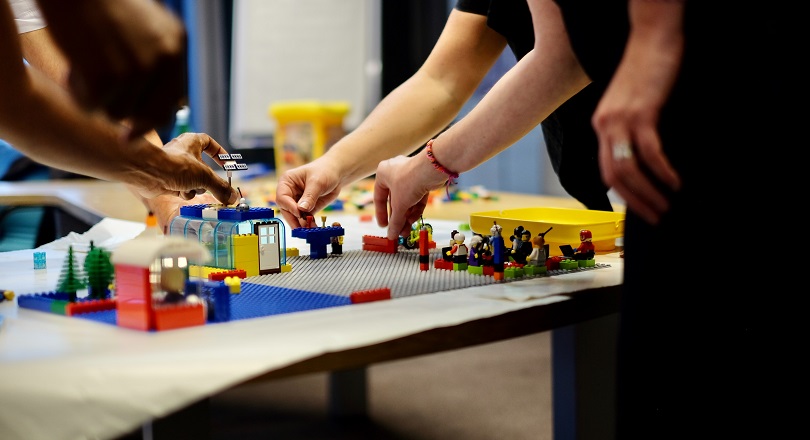
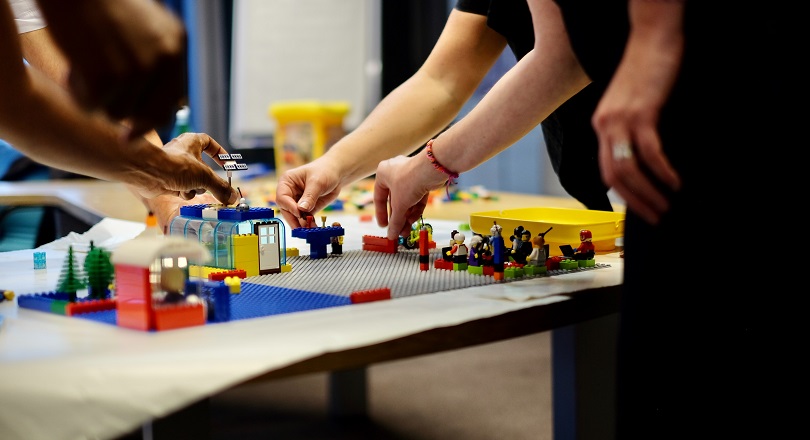
VBA Statements: The Building Blocks of VBA Programs
VBA programs are nothing but a sequence of VBA statements. They are the cornerstone of VBA programs. Here, we explore these vital elements of VBA coding.
![The While – Wend statement’s syntax. Square brackets, [ ], indicate optional items.](https://masterofficevba.com/wp-content/uploads/2021/03/While_Wend_Statement_Syntaxnew.png)
The While – Wend Statement | VBA Iteration Statements
VBA programs often include repetitive execution paths. The While – Wend statement enables such loops. Here, we explore this crucial code construct in-depth.
![The Do – Loop Until statement’s syntax. Square brackets, [ ], indicate optional items while vertical bars, |, indicate mutually exclusive items.](https://masterofficevba.com/wp-content/uploads/2021/03/Do_Loop_Until_Statement_Syntaxnew.png)
The Do – Loop Until Statement | VBA Iteration Statements
VBA programs often include repetitive execution paths. The Do – Loop Until statement enables such loops. Here, we explore this vital code construct in-depth.
![The Do – Loop While statement’s syntax. Square brackets, [ ], indicate optional items while vertical bars, |, indicate mutually exclusive items.](https://masterofficevba.com/wp-content/uploads/2021/03/Do_Loop_While_Statement_Syntaxnew.png)
The Do – Loop While Statement | VBA Iteration Statements
VBA programs often include repetitive execution paths. The Do – Loop While statement enables such loops. Here, we explore this crucial code construct in-depth.
![The Do Until – Loop statement’s syntax. Square brackets, [ ], indicate optional items.](https://masterofficevba.com/wp-content/uploads/2021/03/Do_Until_Loop_Statement_Syntaxnew.png)
The Do Until – Loop Statement | VBA Iteration Statements
VBA programs often include repetitive execution paths. The Do Until – Loop statement enables such loops. Here, we explore this vital code construct in-depth.
![The Do While – Loop statement’s syntax. Square brackets, [ ], indicate optional items.](https://masterofficevba.com/wp-content/uploads/2021/03/Do_While_Loop_Statement_Syntaxnew.png)
The Do While – Loop Statement | VBA Iteration Statements
VBA programs often include repetitive execution paths. The Do While – Loop statement enables such loops. Here, we explore this crucial code construct in-depth.
![The For Each – Next statement’s syntax. Square brackets, [ ], indicate optional items.](https://masterofficevba.com/wp-content/uploads/2021/03/For_Each_Next_Statement_Syntaxnew.png)
The For Each – Next Statement | VBA Iteration Statements
VBA programs often have repetitive execution paths. The For Each – Next statement enables such loops. Here, we explore this vital code construct in-depth.
![The For – Next statement’s syntax. Square brackets, [ ], indicate optional items.](https://masterofficevba.com/wp-content/uploads/2021/03/For_Next_Statement_Syntaxnew.png)
The For – Next Statement | VBA Iteration Statements
VBA programs often have repetitive execution paths. The For – Next statement enables such VBA loops. Here, we explore this crucial code construct in-depth.
![The On Error and Resume statements’ syntax. Square brackets, [ ], vertical bars, |, and curly braces, {}, indicate optional items, mutually exclusive items, and mere item groupings, respectively.](https://masterofficevba.com/wp-content/uploads/2021/03/On_Error_and_Resume_Statements_Syntaxnew.png)
The On Error statement | VBA Jump Statements
Here, you’ll explore the On Error Statement which transfers control to error-handling subroutines when a runtime error occurs.
![The On -GoSub statement’s syntax. Square brackets, [ ], vertical bars, |, and curly braces, {}, indicate optional items, mutually exclusive items, and mere item groupings, respectively.](https://masterofficevba.com/wp-content/uploads/2021/03/On_GoSub_Statement_Syntaxnew.png)
The On – GoSub statement | VBA Jump Statements
Here, you’ll explore the On – GoSub statement which conditionally transfers control between a code-line and a subroutine in the same scope.
![The GoSub – Return statement’s syntax. Square brackets, [ ], vertical bars, |, and curly braces, {}, indicate optional items, mutually exclusive items, and mere item groupings, respectively.](https://masterofficevba.com/wp-content/uploads/2021/03/GoSub_Return_Statement_Syntaxnew.png)
The GoSub – Return statement | VBA Jump Statements
Here, you’ll explore the GoSub – Return statement which unconditionally transfers control between a code-line and a subroutine in the same scope.
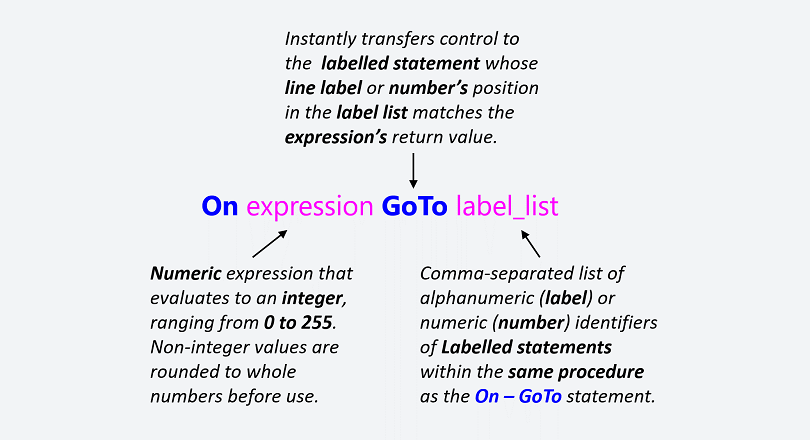
The On – GoTo statement | VBA Jump Statements
Here, you’ll explore the On – GoTo statement which conditionally transfers control to any labelled statement within the same scope (i.e., procedure).
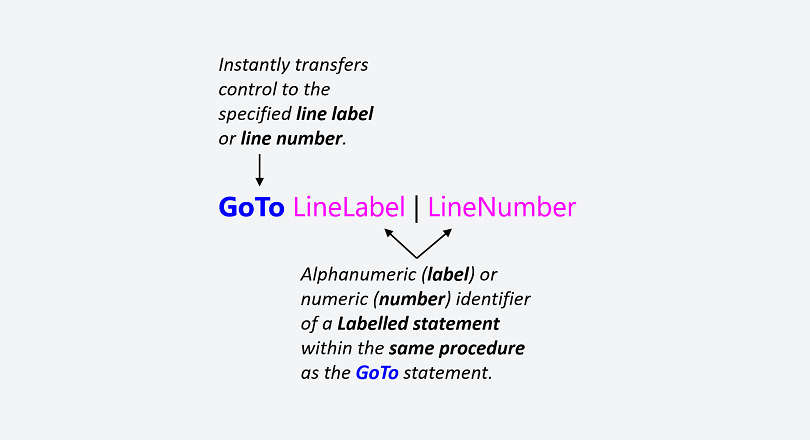
The GoTo statement | VBA Jump Statements
Here, you’ll explore the GoTo statement which unconditionally transfers control to any labelled statement in the same scope (i.e., procedure).
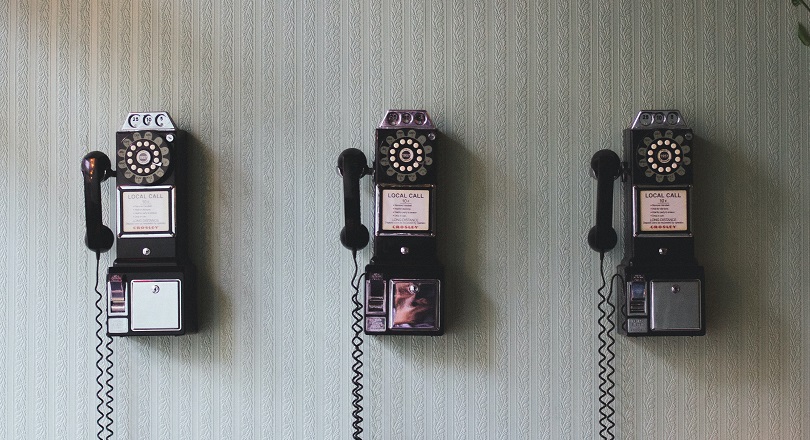
The Call Statement | VBA Jump Statements
Here, you’ll explore the Call statement which transfers control between procedures and DLLs. It is a VBA jump statement for non-sequential code execution.
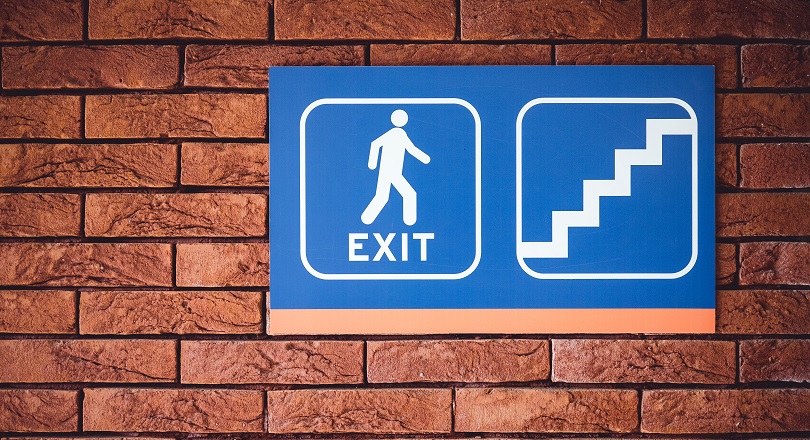
The Exit Statement | VBA Jump Statements
Here, you’ll explore the Exit statement which instantly leaves a block. It is one of several VBA jump statements that enables non-sequential code execution.
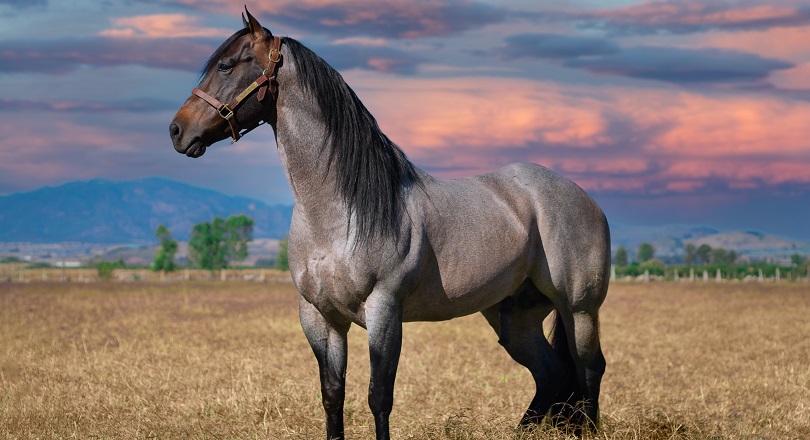
Expression Statements: VBA’s Workhorse
Expression statements do the heavy lifting in VBA. That is because they are prevalent in typical VBA programs. This article introduces these workhorses.
![The Select Case statement’s syntax. Square brackets, [ ], indicate optional items.](https://masterofficevba.com/wp-content/uploads/2021/03/Select_Case_Statement_Syntaxnew.png)
The Select Case Statement | VBA Selection Statements
Most VBA programs feature decision-based execution paths. The Select Case statement is one of two VBA decision statements. So, its exploration is vital.
![The If–Then–[ElseIf]–[Else] statement’s syntax. Square brackets, [ ], indicate optional items.](https://masterofficevba.com/wp-content/uploads/2021/03/If_Then_ElseIf_Else_Statement_Syntaxnew.png)
The If Statement | VBA Selection Statements
Most VBA programs feature decision-based execution paths. The If statement is one of two VBA decision statement. So, its exploration is vital.
![Declaring Enumerations - the Enum statement’s syntax. Square brackets, [ ], indicate optional items while vertical bars, |, indicate mutually exclusive items.](https://masterofficevba.com/wp-content/uploads/2021/03/Enum_Statement_Syntaxnew.png)
The Enum Statement | VBA Declaration Statements
The Enum statement declares VBA enumerations or Enums. Enums are widely used in VBA programs, so it’s useful exploring their declaration, as you’ll do here.
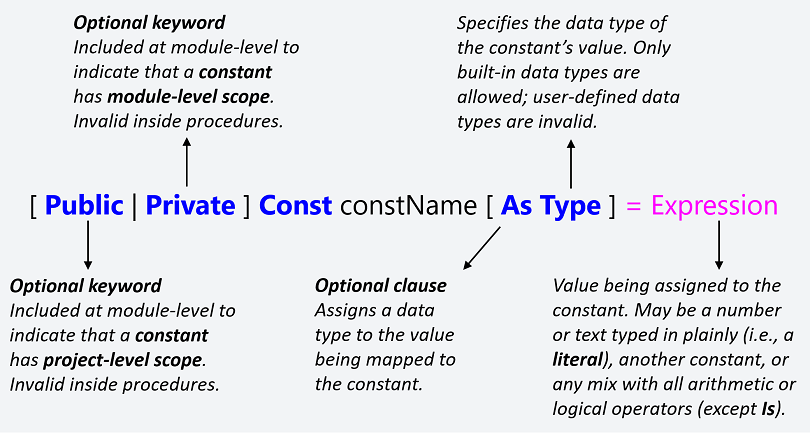
The Const Statement | VBA Declaration Statements
The Const statement declares constants in VBA. Constants are prevalent in VBA programs, so it is useful to explore their declaration, as you’ll do here.
![Declaring Variables - the Dim statement’s syntax. Square brackets, [ ], indicate optional items while vertical bars, |, indicate mutually exclusive items.](https://masterofficevba.com/wp-content/uploads/2021/03/Dim_Statement_Syntaxnew.png)
The Dim Statement | VBA Declaration Statements
The Dim statement declares VBA variables and objects. It is ubiquitous in most VBA programs, so it is worthwhile exploring it in-depth as you’ll do here.
![Declaring Function Procedures - the Function statement’s syntax. Square brackets, [ ], indicate optional items while vertical bars, |, indicate mutually exclusive items.](https://masterofficevba.com/wp-content/uploads/2021/03/Function_Statement_Syntaxnew.png)
The Function Statement | VBA Declaration Statements
The Function statement declares VBA functions. All executable VBA code is in such procedures, so this post’s detailing of the Function statement is vital.
![Declaring Sub Procedures - the Sub statement’s syntax. Square brackets, [ ], indicate optional items while vertical bars, |, indicate mutually exclusive items.](https://masterofficevba.com/wp-content/uploads/2021/03/Sub_Statement_Syntaxnew1.png)
The Sub Statement | VBA Declaration Statements
The Sub statement declares VBA subroutines. All executable VBA code is in such procedures, so, exploring the Sub statement, as you’ll do here, is vital.
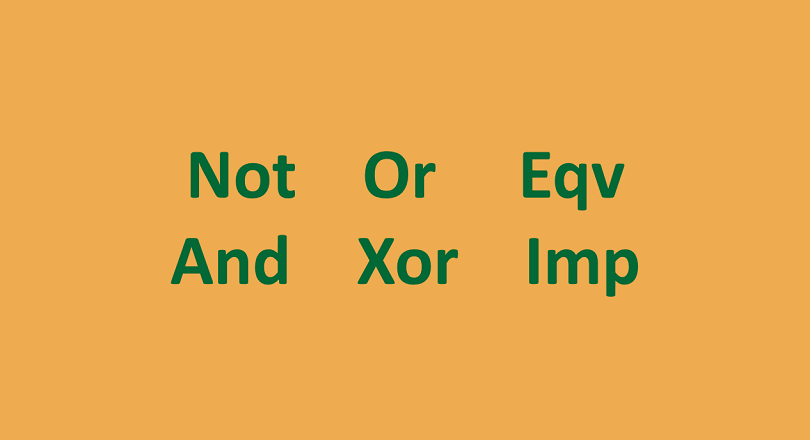
Logical Operators in VBA
VBA logical operators – symbols that instruct the compiler to perform logical operations like conjunction and disjunction – are explored herein.
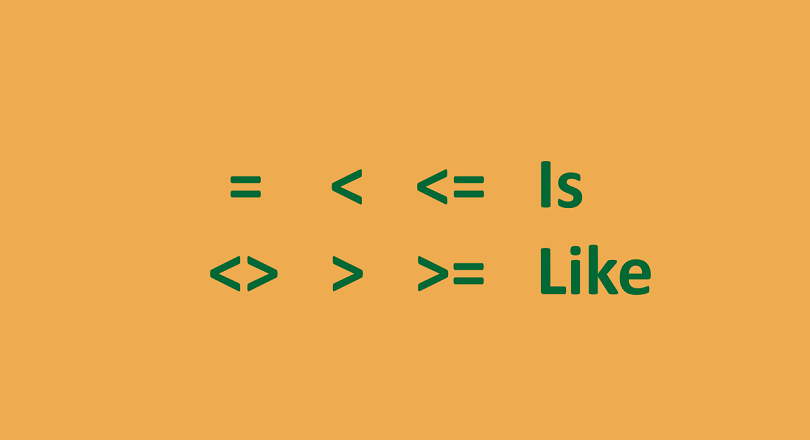
Comparison Operators in VBA
VBA comparison operators – symbols that instruct the compiler to perform relational, object equality, and string match operations- are explored herein.
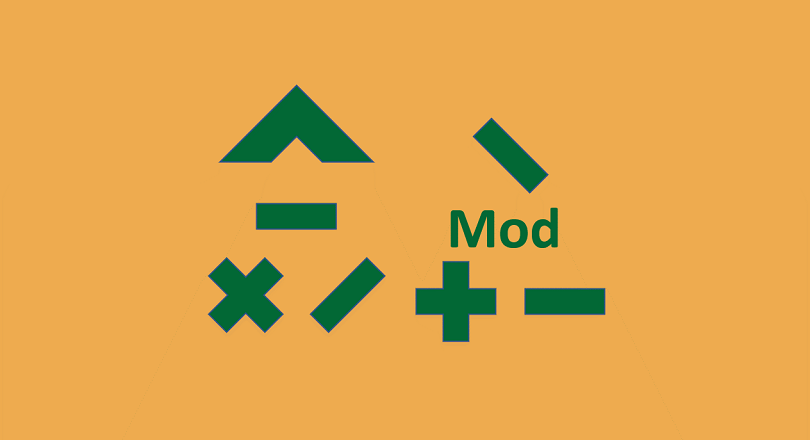
Arithmetic Operators in VBA
VBA arithmetic operators – symbols that instruct the compiler to perform basic math (add, divide, powers, modulus, etc.) – are explored herein.
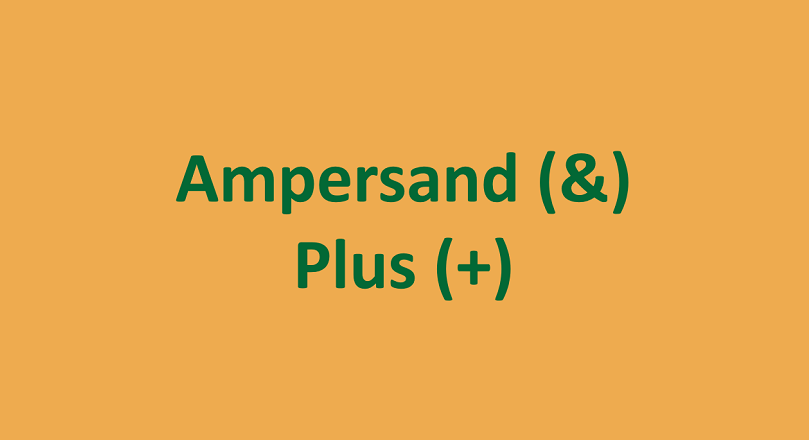
Concatenation Operators in VBA
VBA concatenation operators – symbols that instruct the compiler to join two String operands together – are explored herein.
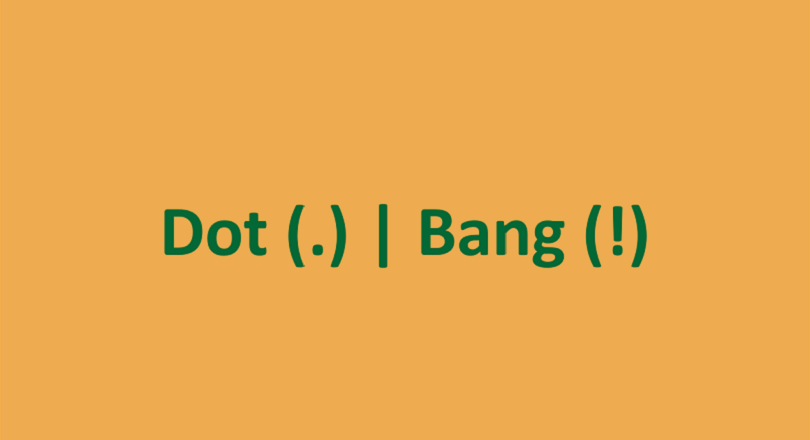
Member Access Operators in VBA
VBA member access operators – symbols that ease the referencing of class or object members (i.e., properties, methods, or events) – are explored herein.
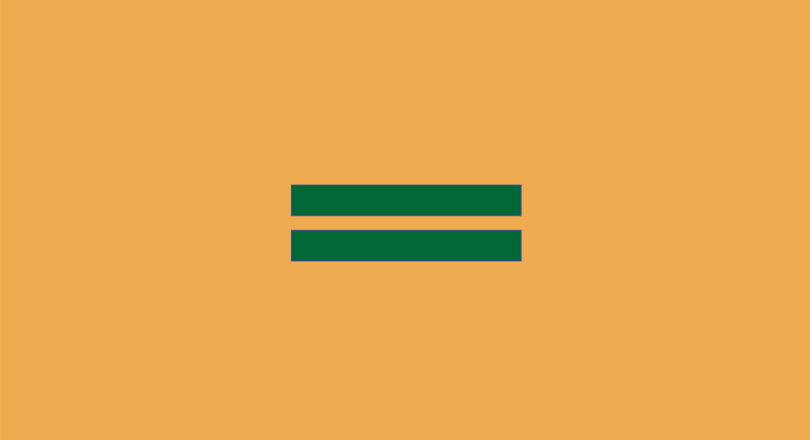
Assignment Operator in VBA
The VBA assignment operator, a symbol that tells the compiler to store the value of the operand on its right in the operand on its left, is explored herein.
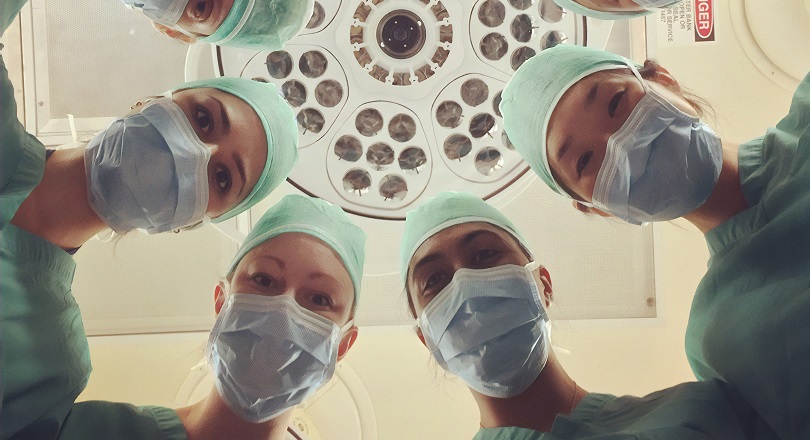
VBA Operators and Precedence
VBA operators – special tokens that instruct the compiler to perform operations on values or value-holding program identifiers – are introduced herein.
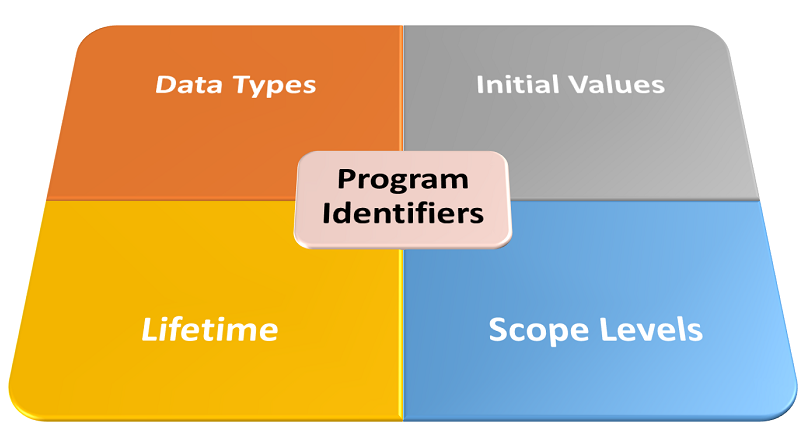
VBA Program Identifiers: Data Type, Scope, and Lifetime
VBA programs often feature named discrete entities called identifiers (e.g., variables). Here, we get familiar with these vital program elements, their attributes, and the rules for legally naming them in VBA.
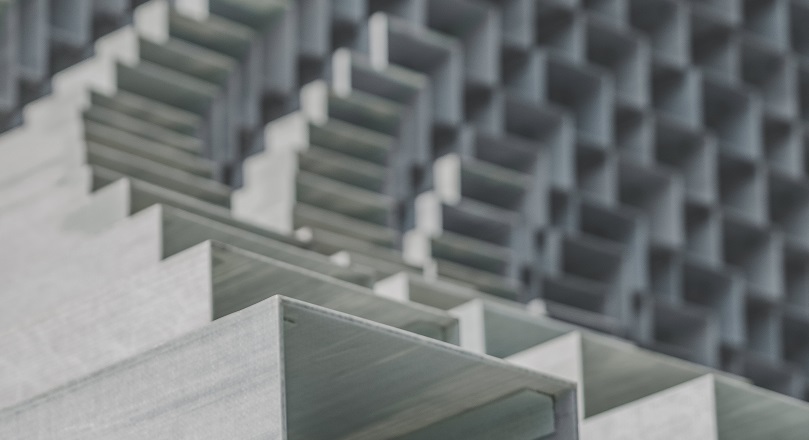
Customizing Projects using the Project Properties Dialog Box
Every developer has IDE preferences for an efficient workflow. Here, we explore VBA project customization with the Project Properties dialog box.
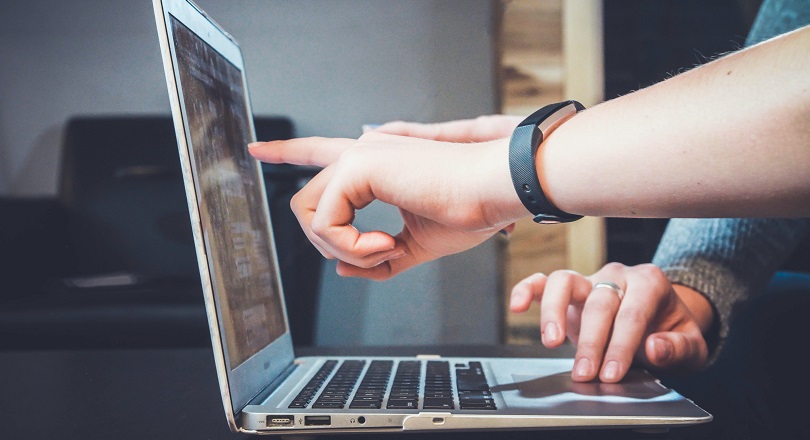
The Options Dialog Box path to Customizing the VBA Editor
Every developer has IDE preferences for an efficient workflow. Here, we explore Excel VBA editor customization available in the Options dialog box.
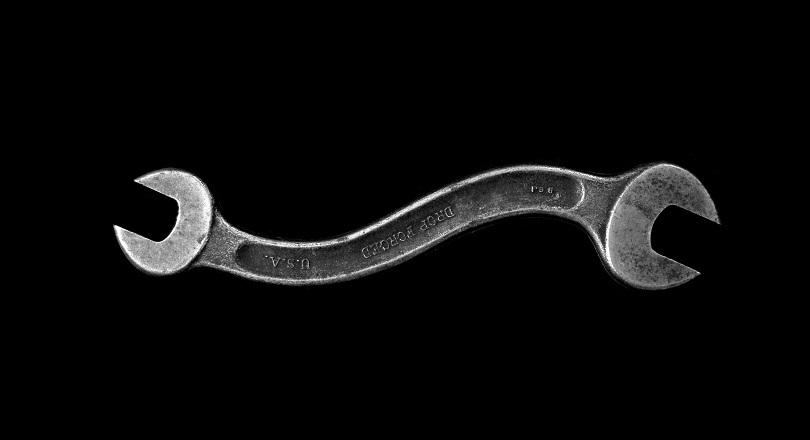
VBA Editor Customization: Menus, Toolbars and Toolbox
Every developer has IDE preferences for an efficient workflow. Here, we explore Excel VBA editor customization for menu, toolbar and userform toolbox.
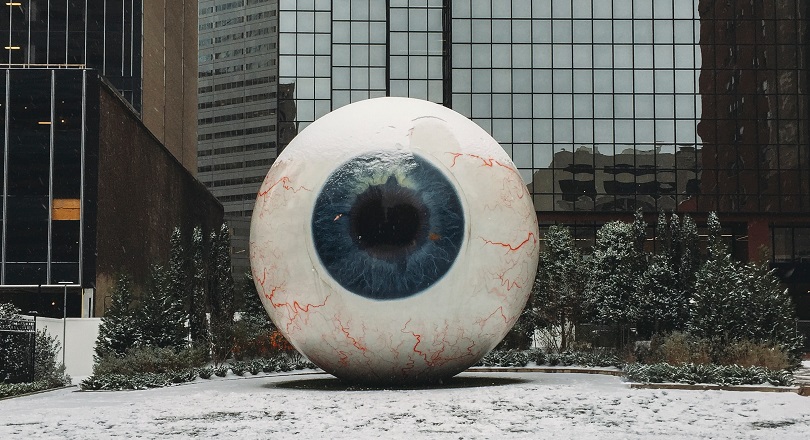
Getting Started with the Watch Window
The Excel VBA coding dojo, the VBA Editor, has many useful windows. Here, you’ll explore its Watch window which lets you statically track variable values
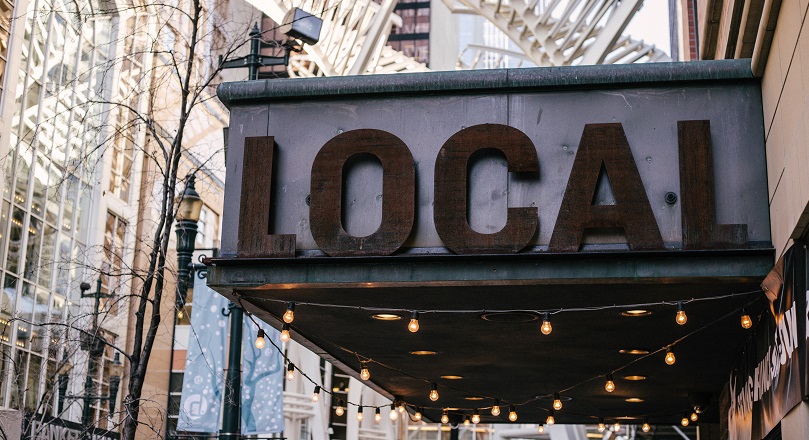
Getting Started with the Locals Window
The Excel VBA coding dojo, the VBA Editor, has many useful windows. Here, you’ll explore its Locals window which lets you dynamically track variable values.
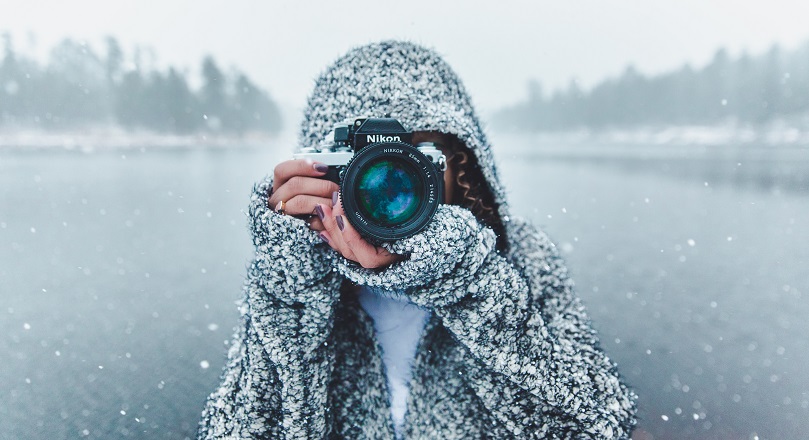
Exploring the VBA Editor’s Immediate Window
The Excel VBA coding dojo, the VBA Editor, has a myriad of very useful windows. Here, you’ll explore its Immediate Window which takes code snapshots.
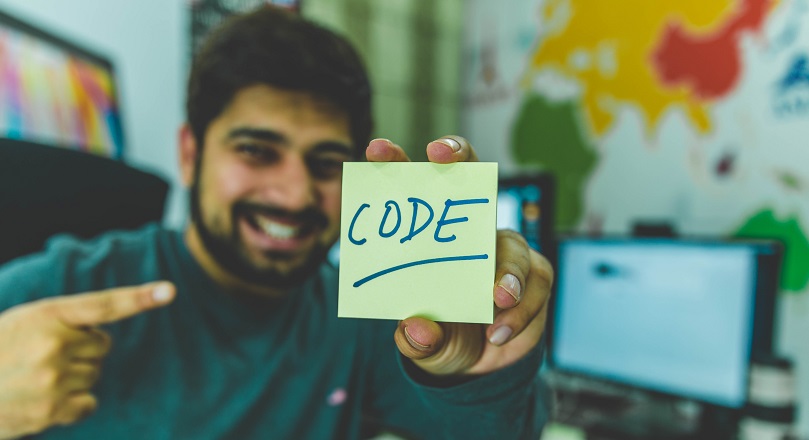
Exploring the VBA Editor’s Code Window
The Excel VBA coding dojo, the VBA Editor, has a myriad of very useful windows. Here, you’ll explore its Code Window and its glut of features.
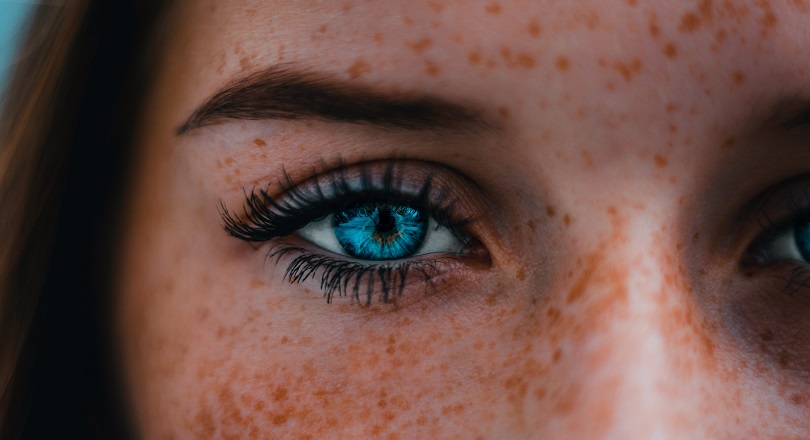
Getting Started with the Properties Window
The Excel VBA coding dojo, the VBA Editor, has a myriad of very useful windows. Here, you’ll explore its Properties Window.
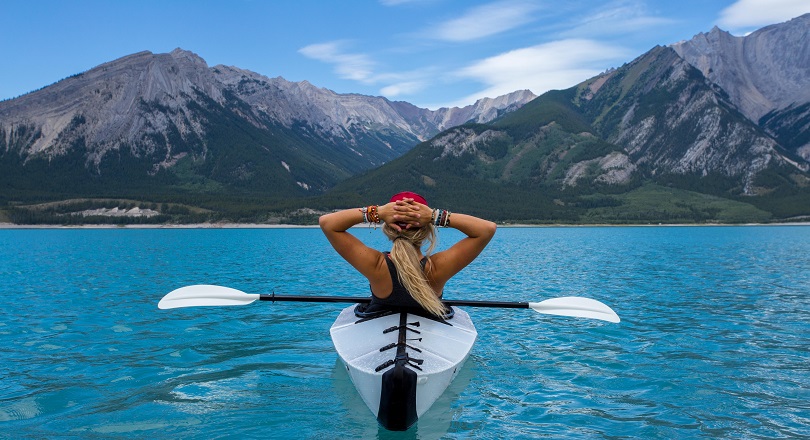
Getting Started with the Project Explorer
To master Excel VBA you’ll have to get comfy in its coding dojo, the VBA Editor. Here, you’ll explore its essential navigation tool, the Project Explorer.
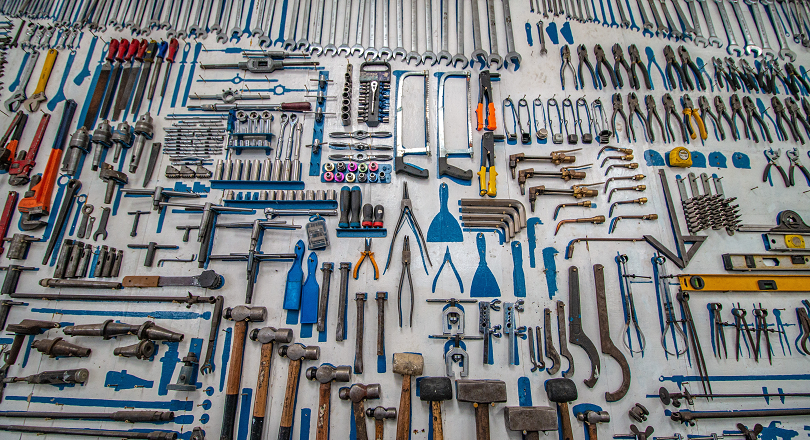
Exploring each VBA Editor Toolbar in Excel
To master Excel VBA you’ll have to get comfy in its coding dojo, the VBA Editor. Here, you’ll take a deep-dive into each VBA editor toolbar and its icons.
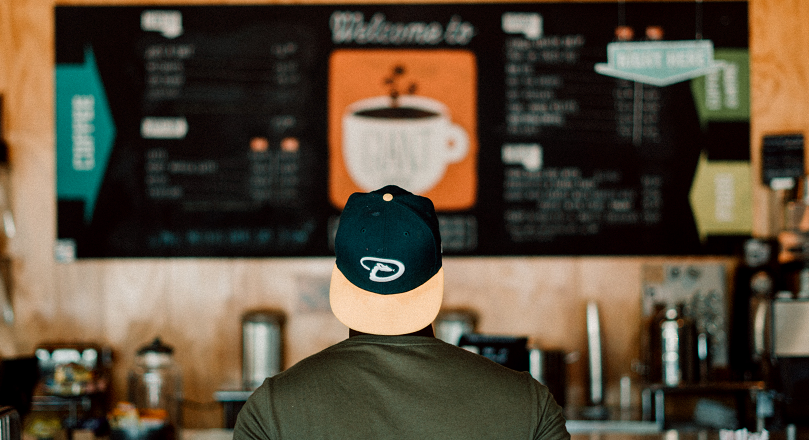
Exploring each VBA Editor Menu in Excel
To master Excel VBA you’ll have to get comfy in its coding dojo, the VBA Editor. Here, you’ll delve into the VBA Editor Menu Bar and its trove of menus.
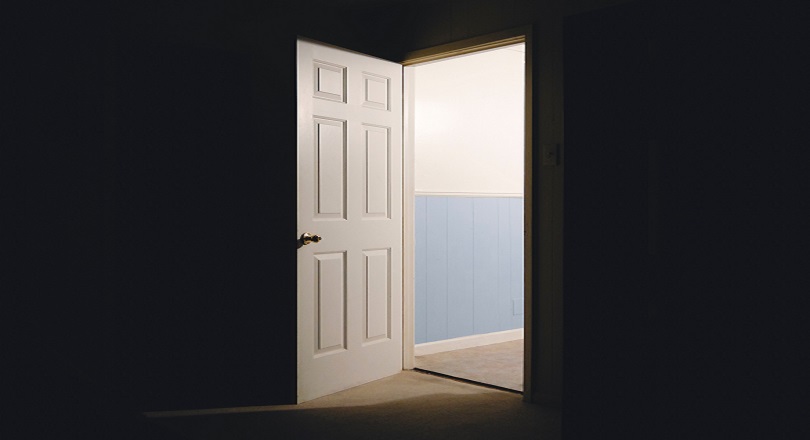
How to Open the VBA Editor in Excel
To master Excel VBA you’ll have to get comfy in its coding dojo, the VBA Editor. Here, you’ll learn how to open the VBA editor using several methods.
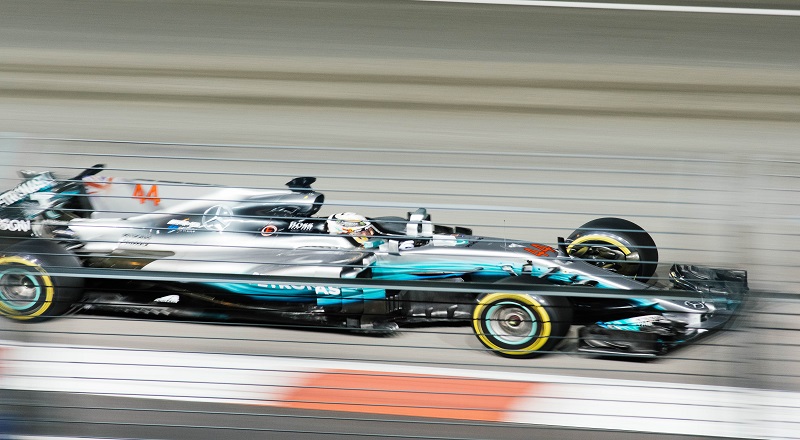
Efficient Excel VBA Code: Best Practices
Learn guidelines for writing efficient Excel VBA code, sure to help you build Excel VBA applications that do more with less.
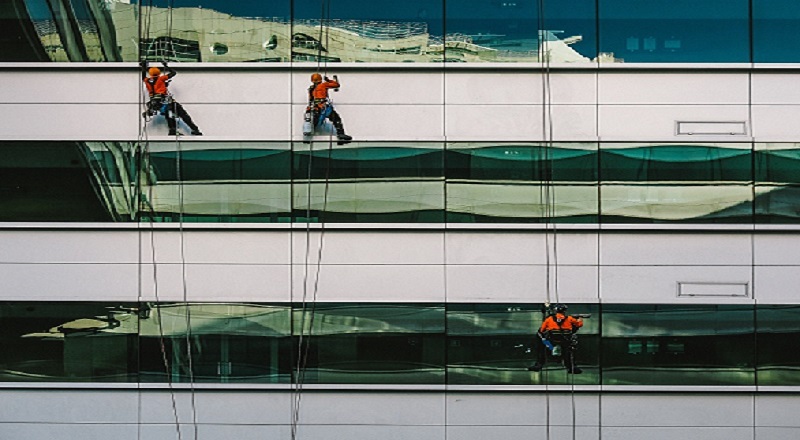
Maintainable Excel VBA Code: Best Practices
Learn guidelines for maintainable Excel VBA code that will help you build Excel VBA applications that are easier to edit or adapt.
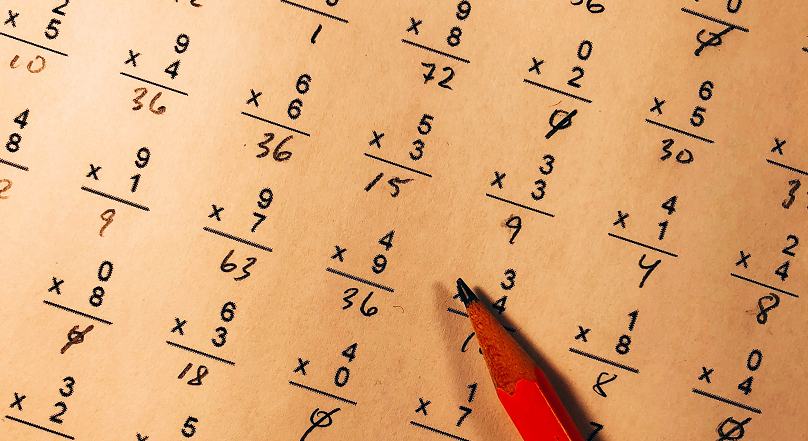
Reliable Excel VBA Code: Best Practices
Learn guidelines for writing reliable Excel VBA code, guaranteed to help you build Excel VBA applications that ship with zero unhandled bugs.
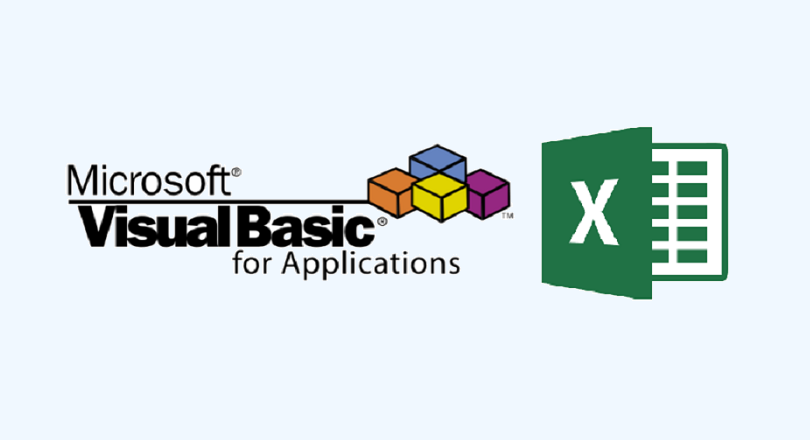
Best Practices for Excel VBA Code
Access a broad collection of Excel VBA programming best practices and tips to boost your Excel VBA code’s quality.
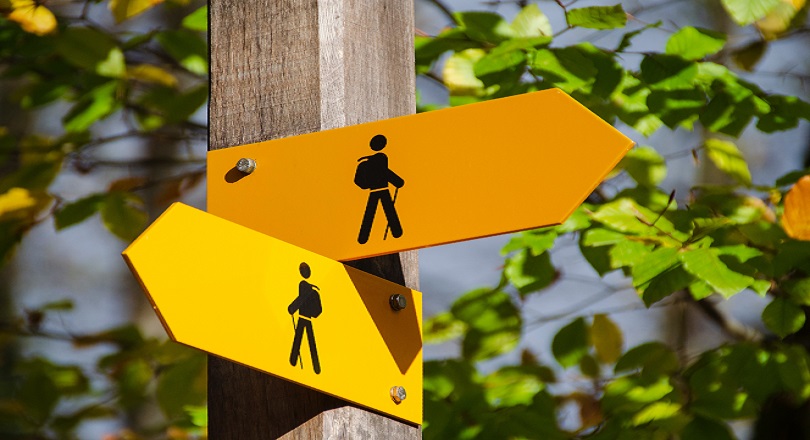
Learn Excel VBA? Top Reasons Why You Should
Since Microsoft dropped VB6 IDE support, ‘VBA is dead’ claims abound. Well, is it? No! Should you learn Excel VBA? Yes! Here, I explain why and more.